To create a new xUnit test project, you can run the dotnet new xunit command, and the CLI does the job for you by creating a project containing a UnitTest1 class. That command does the same as creating a new xUnit project from Visual Studio.For unit testing projects, name the project the same as the project you want to test and append .Tests to it. For example, MyProject would have a MyProject.Tests project associated with it. We explore more details in the Organizing your tests section below.The template already defines all the required NuGet packages, so you can start testing immediately after adding a reference to your project under test.
You can also add project references using the CLI with the dotnet add reference command. Assuming we are in the ./test/MyProject.Tests directory and the project file we want to reference is in the ./src/MyProject directory; we can execute the following command to add a reference:
dotnet add reference ../../src/MyProject.csproj.
Next, we explore some xUnit features that will allow us to write test cases.
Key xUnit features
In xUnit, the [Fact] attribute is the way to create unique test cases, while the [Theory] attribute is the way to make data-driven test cases. Let’s start with facts, the simplest way to write a test case.
Facts
Any method with no parameter can become a test method by decorating it with a [Fact] attribute, like this:
public class FactTest
{
[Fact]
public void Should_be_equal()
{
var expectedValue = 2;
var actualValue = 2;
Assert.Equal(expectedValue, actualValue);
}
}
You can also decorate asynchronous methods with the fact attribute when the code under test needs it:
public class AsyncFactTest
{
[Fact]
public async Task Should_be_equal()
{
var expectedValue = 2;
var actualValue = 2;
await Task.Yield();
Assert.Equal(expectedValue, actualValue);
}
}
In the preceding code, the highlighted line conceptually represents an asynchronous operation and does nothing more than allow using the async/await keywords.When we run the tests from Visual Studio’s Test Explorer, the test run result looks like this:
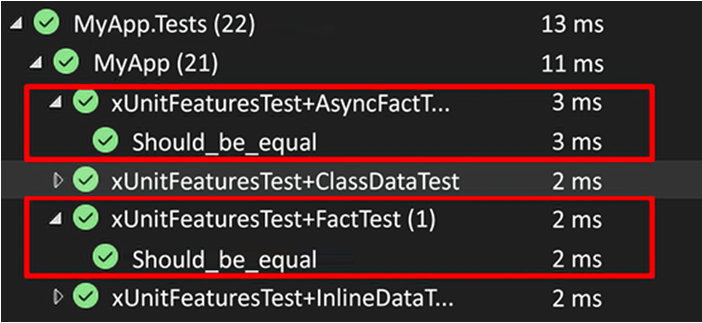
Figure 2.3: Test results in Visual Studio
You may have noticed from the screenshot that the test classes are nested in the xUnitFeaturesTest class, part of the MyApp namespace, and under the MyApp.Tests project. We explore those details later in the chapter.Running the dotnet test CLI command should yield a result similar to the following:
Passed!
– Failed: 0, Passed: 23, Skipped: 0, Total: 23, Duration: 22 ms – MyApp.Tests.dll (net8.0)
As we can read from the preceding output, all tests are passing, none have failed, and none were skipped. It is as simple as that to create test cases using xUnit.
Learning the CLI can be very helpful in creating and debugging CI/CD pipelines, and you can use them, like the dotnet test command, in any script (like bash and PowerShell).
Have you noticed the Assert keyword in the test code? If you are not familiar with it, we will explore assertions next.